
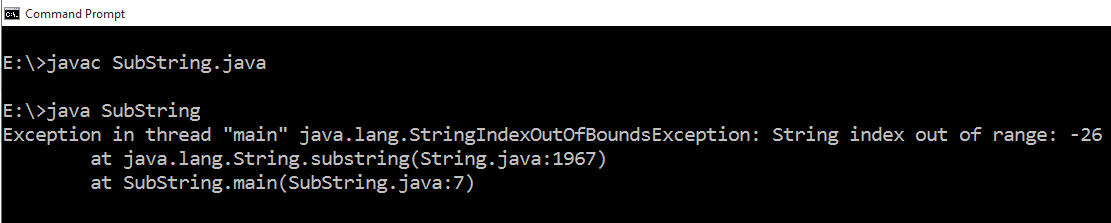
The code could be simplified, but I've intentionally made it verbose so you can easily step through it in a debugger. For consistency with item 2, negative return values also start counting down starting with -1 as the index of the last character.
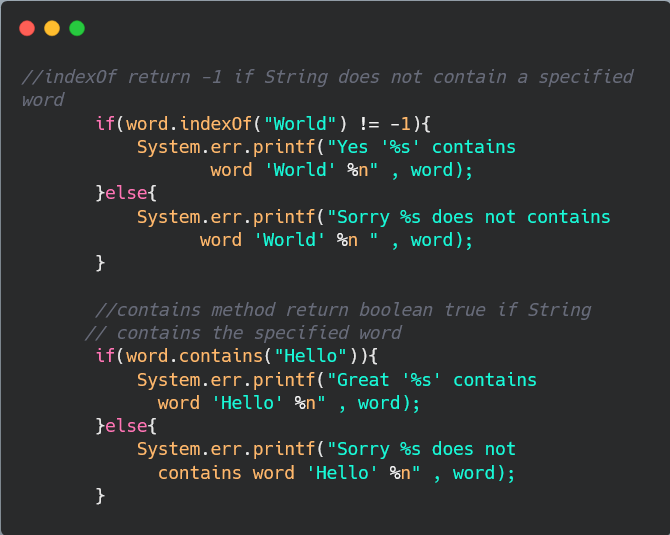
For that reason, we use -1 to refer to the index of the last character, and continue counting backwards from there.
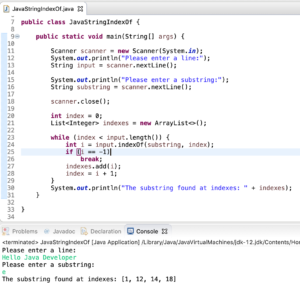
As it turns out, the indexOf implementation that is ultimately called resets a negative fromIndex value to zero: static int indexOf(char source, int sourceOffset, int sourceCount,Ĭhar target, int targetOffset, int targetCount, To answer your question about what happens when you pass a negative number, you can dig into the source code for the String class. This method may be used to trim whitespace (as defined above) from the beginning and end of a string. That's because indexOf (String) is a JVM intrinsic: its Java code is actually replaced by JIT compiler with efficient inline implementation. The indexOfString is slightly faster for direct access, but somewhat slower for indirect. You can also use lastIndexOf(int ch, int fromIndex)if you want to search backwards from a particular index. A String object is returned, representing the substring of this string that begins with the character at index k and ends with the character at index m -that is, the result of this.substring (k, m + 1). As you can see, indexOfChar performs in the same way regardless of direct or indirect access. Then you could subtract that number from the length of the String and negate it, if that's what you really want. LastIndexOf(int ch) will start from the end and search backwards, returning the absolute index of the last occurrence.
